آموزش نحوه نوشتن برنامه ماشین حساب به زبان c
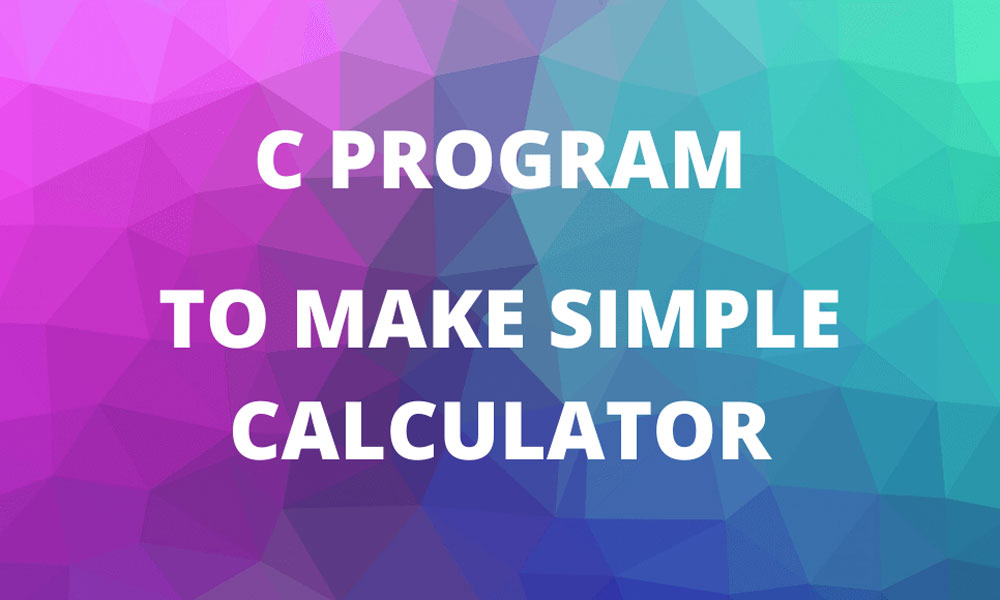
کد برنامه ماشین حساب به زبان c
در این مقاله میخواهیم به نحوه نوشتن کد برنامه ماشین حساب به زبان c بپردازیم.
همانطور که میدانید ماشین حساب نرمافزار سادهای است که برای انجام عمیاتهای مختلف مانند جمع ، تفریق ، ضرب ، تقسیم ،
درصد و … به کارمیرود. ماشین حسابهای پیچیدهتر میتوانند برای محاسبات پیچیدهتر مانند توابع مثلثاتی ، توابع لگاریتم یا توابع
هذلولی مورد استفاده قرار گیرد.
الگوریتم برای نوشتن کد برنامه ماشین حساب به زبان سی
مراحل ایجاد الگوریتم برای نوشتن کد برنامه ماشین حساب به زبان سی:
- قدم اول: متغیرهای n1 ، n2 و … ، res و opt را برای برنامه تعریف کنید. ( به طوری که برنامه برای متغیرهای n مقادیر عددی
دریافت میکند ، متغیر opt برای تعریف نمادهای عملگر به کار میرود و نتایج درمتغیر res ذخیره میشوند. ) - قدم دوم: عملگر را چاپ کند. ( ضرب ، تقسیم ، جمع یا تفریق )
- قدم سوم: عملگر مود نظر انتخاب شود.
- قدم چهارم: دو عدد را به عنوان ورودی n1 و n2 دریافت کند.
- قدم پنجم: عملگر انتخاب شده توسط کاربر را برای متغیرهای ورودی اعمال کند.
- قدم ششم: نتایج را در متغیر res ذخیره کند.
- قدم هفتم: نتیجه را نمایش دهد.
- قدم هشتم: برنامه متوقف شود.
روشهای نوشتن کد برنامه ماشین حساب به زبان c
چندین روش برای نوشتن کد برنامه ماشین حساب به زبان c وجود دارد ولی الگوریتم تمامی آنها مشابه است :
• نوشتن کد برنامه ماشین حساب به زبان c با دستور if else if
• نوشتن برنامه ماشین حساب به زبان c با استفاده از دستور حلقه و سوئیچ do-while
• نوشتن برنامه ماشین حساب به زبان c با استفاده از تابع و دستور سوئیچ
• نوشتن کد برنامه ماشین حساب به زبان c با دستور switch
در این مطلب روش آخر را با یک مثال بررسی خواهیم کرد:
#include <stdio.h> int main() { // declare local variables char opt; int n1, n2; float res; printf (” Choose an operator(+, -, *, /) to perform the operation in C Calculator \n “); scanf (“%c”, &opt); // take an operator if (opt == ‘/’ ) { printf (” You have selected: Division”); } else if (opt == ‘*’) { printf (” You have selected: Multiplication”); } else if (opt == ‘-‘) { printf (” You have selected: Subtraction”); } else if (opt == ‘+’) { printf (” You have selected: Addition”); } printf (” \n Enter the first number: “); scanf(” %d”, &n1); // take fist number printf (” Enter the second number: “); scanf (” %d”, &n2); // take second number switch(opt) { case ‘+’: res = n1 + n2; // add two numbers printf (” Addition of %d and %d is: %.2f”, n1, n2, res); break; case ‘-‘: res = n1 – n2; // subtract two numbers printf (” Subtraction of %d and %d is: %.2f”, n1, n2, res); break; case ‘*’: res = n1 * n2; // multiply two numbers printf (” Multiplication of %d and %d is: %.2f”, n1, n2, res); break; case ‘/’: if (n2 == 0) // if n2 == 0, take another number { printf (” \n Divisor cannot be zero. Please enter another value “); scanf (“%d”, &n2); } res = n1 / n2; // divide two numbers printf (” Division of %d and %d is: %.2f”, n1, n2, res); break; default: /* use default to print default message if any condition is not satisfied */ printf (” Something is wrong!! Please check the options “); } return 0; } |
کد بالا با دستور switch برنامهی ماشین حساب را به زبان c مینویسد که دو عدد را گرفته و میتواند چهار عمل اصلی را برای
آنها اعمال کند. خروجی این برنامه به شکل زیر خواهد بود:
توجه داشته باشید که در دستورالعمل switch حتما باید پایان هر دستور،break باشد.
سورس کد ماشین حساب مهندسی به زبان c++:
با کمک زبان c++ نیز میتوانیم کدی برای ایجاد یک ماشین حساب بنویسیم. الگوریتم کدنویسی مانند گذشته است و این بار هم از دستور switch برای نوشتن برنامه استفاده میکنیم، با این تفاوت که ماشین حساب ایجاد شده علاوه بر چهار عمل اصلی بتواند مربع و جذر عدد ورودی را نیز محاسبه کند.
#include<iostream.h> #include<stdio.h> #include<conio.h> #include<math.h> #include<stdlib.h> void add(); void sub(); void multi(); void division(); void sqr(); void srt(); void exit(); void main() { clrscr(); int opr; // display different operation of the calculator do { cout << “Select any operation from the C++ Calculator” “\n1 = Addition” “\n2 = Subtraction” “\n3 = Multiplication” “\n4 = Division” “\n5 = Square” “\n6 = Square Root” “\n7 = Exit” “\n \n Make a choice: “; cin >> opr; switch (opr) { case 1: add(); // call add() function to find the Addition break; case 2: sub(); // call sub() function to find the subtraction break; case 3: multi(); // call multi() function to find the multiplication break; case 4: division(); // call division() function to find the division break; case 5: sqr(); // call sqr() function to find the square of a number break; case 6: srt(); // call srt() function to find the Square Root of the given number break; case 7: exit(0); // terminate the program break; default: cout <<“Something is wrong..!!”; break; } cout <<” \n——————————\n”; }while(opr != 7); getch(); } void add() { int n, sum = 0, i, number; cout <<“How many numbers you want to add: “; cin >> n; cout << “Please enter the number one by one: \n”; for (i = 1; i <= n; i++) { cin >> number; sum = sum + number; } cout << “\n Sum of the numbers = “<< sum; } void sub() { int num1, num2, z; cout <<” \n Enter the First number = “; cin >> num1; cout << “\n Enter the Second number = “; cin >> num2; z = num1 – num2; cout <<“\n Subtraction of the number = ” << z; } void multi() { int num1, num2, mul; cout <<” \n Enter the First number = “; cin >> num1; cout << “\n Enter the Second number = “; cin >> num2; mul = num1 * num2; cout <<“\n Multiplication of two numbers = ” << mul; } void division() { int num1, num2, div = 0; cout <<” \n Enter the First number = “; cin >> num1; cout << “\n Enter the Second number = “; cin >> num2; while ( num2 == 0) { cout << “\n Divisor canot be zero” “\n Please enter the divisor once again: “; cin >> num2; } div = num1 / num2; cout <<“\n Division of two numbers = ” << div; } void sqr() { int num1; float sq; cout <<” \n Enter a number to find the Square: “; cin >> num1; sq = num1 * num1; cout <<” \n Square of ” << num1<< ” is : “<< sq; } void srt() { float q; int num1; cout << “\n Enter the number to find the Square Root:”; cin >> num1; q = sqrt(num1); cout <<” \n Square Root of ” << num1<< ” is : “<< q; } |
خروجی برنامه ماشین حساب به زبان c++ به صورت زیر خواهد بود: